- Christopher McKinney
- May 27, 2020
- 2 min read
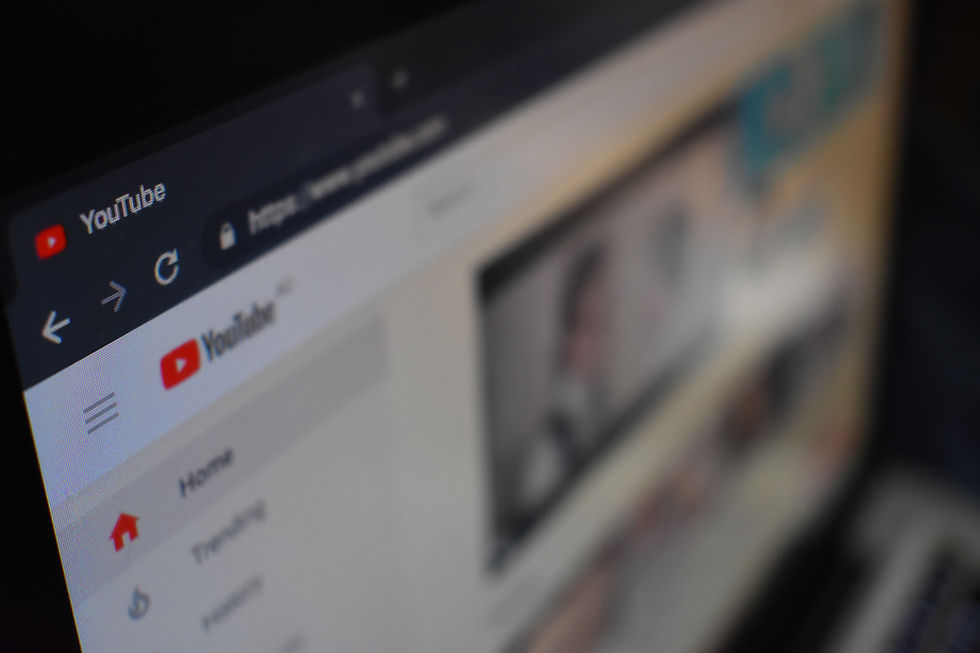
This will be the final blog post on the Circuit Talk website. I hope that all of you viewers have enjoyed the lessons that I have given on how to design circuits, solder, and most importantly, program. As I look back on the progress of my blog, website, and podcasts, I really see how much I have improved in communicating my passions and ideas to a community that is also interested. Since I have been teaching and informing in all of my last blog posts, I thought that I would be appropriate to share the things that I have learned going through this social media process.
The first thing I learned when creating my website is that you want to keep it very minimal and easy to navigate. You don't want to distract your visitors or overwhelm them with too much information. In addition to this, it should also looked really appealing. I was able to make my website appealing by using a good color scheme with the variations of blue and a simple logo.
For social media posts, the best thing to do is post frequently to keep all of your followers updated. This involves letting them know when you release new content, such as blog posts and podcasts on the website. These posts should give a little bit of detail to what the blog/podcast is about.
Finally, for a podcast, it is VERY important that you plan the podcast out beforehand and make a detailed outline of what you are going to talk about. Through the podcasts that I have done. I have realized that trying to talk about a certain topic without an outline is very hard. However, I also recommend not writing word for word what you are going to say. This can make the podcast sound very monotone and uninteresting.
After learning all of these social media and website management skills, I have decided that I am likely going to start my own YouTube channel, going into further detail about all of the circuit projects that I am designing. This will allow me to share my passion with other people that are interested. When I create this channel, I will add the link on this website so it can be accessed. I hope you all enjoyed the blog series and learned something new!